Godot 4 is on its way to the first release candidate. The difference between Godot 3.x and Godot 4 is huge. While there are already various tutorials on many of the changes, it seems to me that the area of network programming has been neglected and that although, or maybe because, there have been a lot of changes there as well. In addition, I’m particularly interested in this part, because so far almost only pure multiplayer games have managed to fascinate me for a long time and I can use my professional experience in this area.
Since the topic is so huge that a book could probably be written about it, I will cover it as part of a tutorial series. The following list shows all articles of this series that have been published so far:
- Networking tutorial 1: General information and overview
- Networking tutorial 2: The "walking skeleton"
- Networking tutorial 3: Login 1 - The game client
- Networking tutorial 4: Login 2 - gateway and authentication server
- Networking tutorial 5: Login 3 - world server
- Networking tutorial 6: Encrypted connections using DTLS
Overview
This tutorial series is intended to get you started with network programming using Godot 4. For this reason, everything created in this series will be created with the current Godot 4 version, even though this may not necessarily be the case in reality, as there are better technologies for certain tasks. But before we get into the practice in the next article, I’d like to first talk about some subjects that influence the chosen architecture, and then present the architecture I’m targeting.
If you are thinking about the topic of server architecture for pure multiplayer games without any previous experience, you will probably come up with such an idea first:
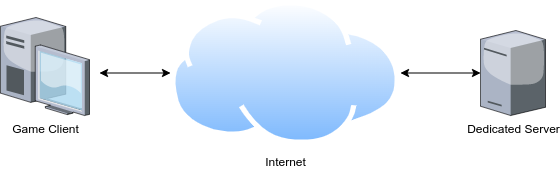
Game clients can communicate directly with the single dedicated game server via the Internet.
It is possible that in these initial considerations it is still in mind that the dedicated server may still need a database, but this is currently only a minor detail. There are certainly many problems with a single dedicated server, but in my opinion the biggest ones are:
- Security
- Scalability
The server architecture for pure multiplayer games must perform at least two tasks: Authenticate new clients and then handle game-relevant communication with all clients. Authentication requires user data, which should not fall into the wrong hands under any circumstances, but in this case are located on a server that is directly connected to the Internet. Especially since access cannot be restricted to certain IP addresses, as this would turn away potential players. We don’t even have to start talking about non-availability in this scenario. When the server is down, neither multiplayer is available, nor are players able to change their user data.
The number of possible players is generally dependent on various factors, which I will discuss in more detail in the next section, but a certain upper limit is omnipresent. So if you want to move the limit up, you will need several servers, and not every server should store all user data.
Potential bottlenecks
There are several factors that influence the number of simultaneously connected players per server:
- Maximum number of concurrent connected players
- Requests per second (RPS)
- Number of players that can be reasonably fit on the game world/map
When using ENet with Godot, there is an upper limit of 4095 simultaneous connections, which means that more players per server are not possible for now. If you still want to support more than ~4k players simultaneously in a game world, you have to distribute the game world over several servers. To do this, the game world is divided into zones and these zones are distributed to the servers. Optimally, this distribution is done dynamically at runtime depending on the respective workload to save resources. But the already high complexity increases significantly due to the distribution to several servers, because solutions have to be developed to enable players to change servers, e.g. when changing zones, in the best case without the players noticing this. But the topic is so complex that it justifies its own articles.
The required number of requests per second (RPS) depends on the selected genre. In fast and interaction-rich genres such as first-person shooters, it is essential to have as many RPS as possible so that all game clients receive their necessary game data (e.g. own position, positions of the other players, positions of “visible” opponents) as quickly as possible in order to display them almost without delay so that the player can react. For games like FarmVille it is also sufficient to have only a few RPS, because on the one hand the multiplayer is used only little as far as I know and on the other hand it is not so relevant for the game, where exactly visitors of the own farm are located. Of course, the number of RPS that can be realistically achieved also depends on how complex the server-side calculations are and how much computing capacity is available.
To create a good gaming experience for players, the number of players should be appropriate for the map size, genre, and core game mechanics. For competitive games, the map size should be large enough that opposing players (teams) do not spawn right next to each other, but small enough that opposing players (teams) still meet in a timely manner. In Hunt:Showdown, a core game mechanic is that actually everything in the game world makes noise that can be well localized thanks to 3D Spatial Audio, so many players try to stay stealthy for as long as possible. To account for this game mechanic, the map is very sparsely “populated” by players with 1 square kilometer for a maximum of 12 players divided into an average of 6 teams. In (M)MORPGs, the world is usually designed for hundreds to thousands of players, but despite this, there are always situations where certain areas are completely overrun, so that questing is then unthinkable. Anyone who wanted to play when a WoW expansion launched knows what I mean by that.
The targeted architecture for the tutorial
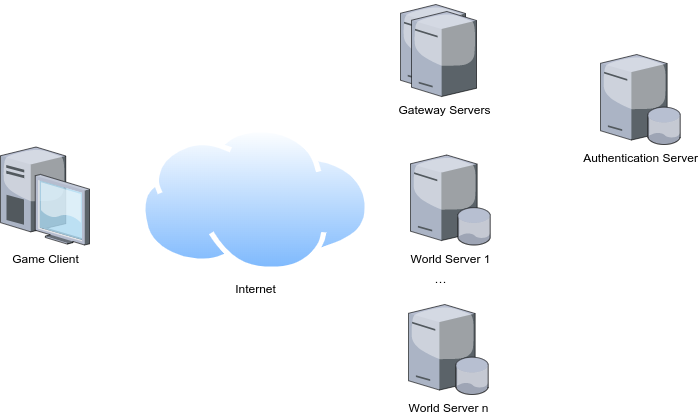
The game clients obtain a token from the Authentication Server via the gateway, which they need to establish a connection to the World Server.
The figure illustrates the architecture that will be created in the course of this tutorial series. It consists of three different types of servers:
- Gateway Server
- Protects the Authentication Server from direct access via internet and forwards only valid requests to the Authentication Server.
- Authentication Server
- Has access to the user data to validate login data and issues a token to access the World Server if the login data is valid.
- World Server
- Validates issued tokens and provides the actual game. In this example, each world server has its own player data, i.e. it is a game with persistent data, e.g. an (M)MORPG.
Besides the architecture, you can also see the communication in the figure:
- the game client sends the login data to one of the gateway servers
- the gateway server forwards the login data to the authentication server, which then verifies them
- the result is sent to the gateway server:
- in the positive case a token
- in the negative case, an error code
- the gateway server forwards the result to the game client
- if the game client has received a token, it sends the token to the desired world server
- the world server validates the token and if it is valid, the actual game starts.
In my opinion, this architecture forms a good way to learn and understand network programming with Godot 4. Your own game will certainly have different requirements, but this gives you a good basis to then adapt it to your own needs.